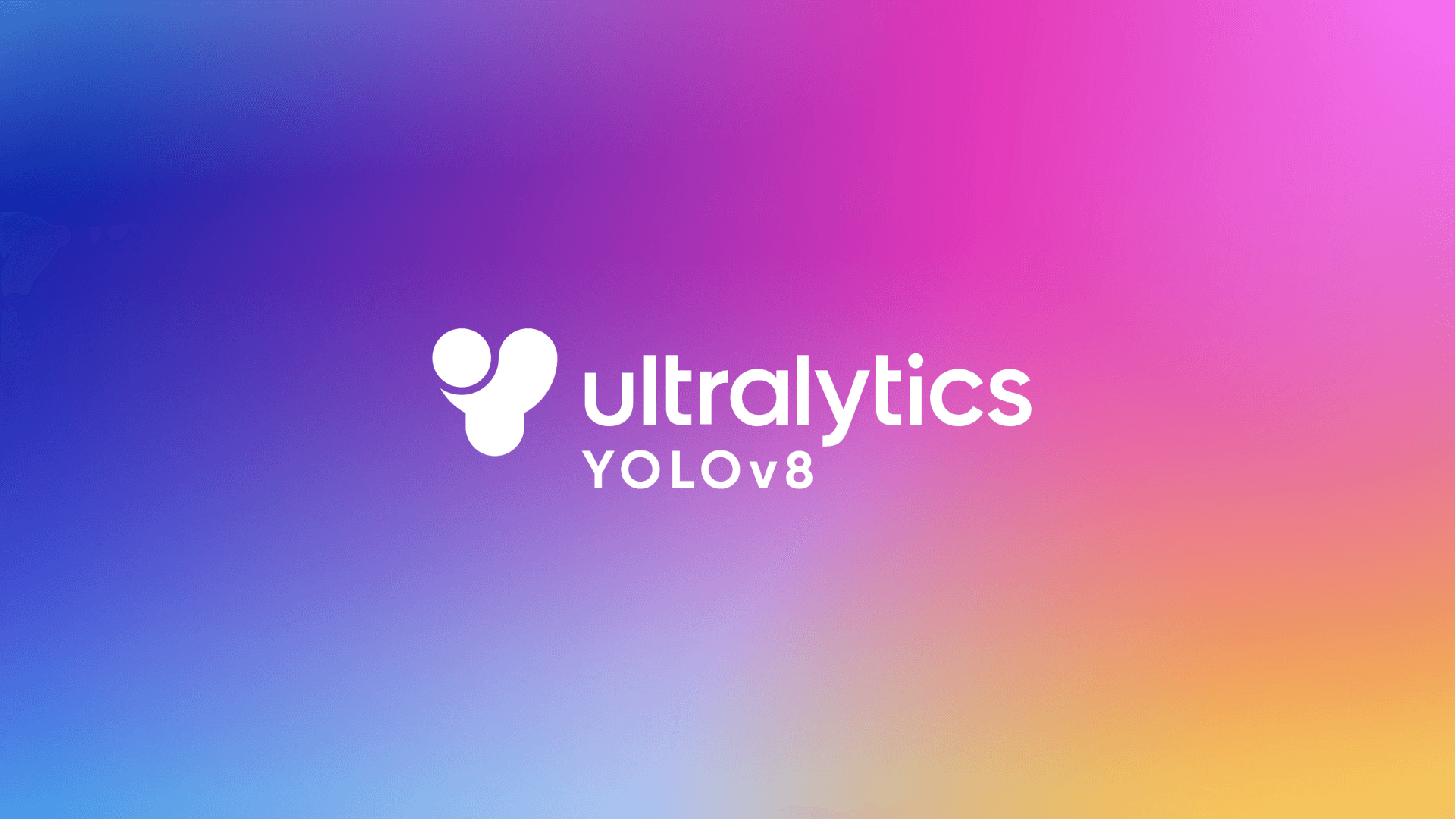
2024. 9. 6.
Introduction
Object detection is a crucial task in computer vision, and YOLOv8 is at the forefront of this technology. In this blog post, we'll explore how to implement YOLOv8 object detection on mobile devices using ZETIC.MLange, a powerful framework for on-device AI applications. After this post you can make your own on-device object detection app utilizing Mobile NPUs.
What is YOLOv8?
YOLOv8 is the latest iteration of the YOLO (You Only Look Once) family of real-time object detection and image segmentation models. Developed by Ultralytics, YOLOv8 offers state-of-the-art performance for various computer vision tasks.
Official YOLOv8 Document page by Ultrylytics: link
What is ZETIC.MLange?: Bringing AI to Mobile devices
ZETIC.MLange is a On-device AI framework that enables developers to deploy complex AI models, like YOLOv8, on mobile devices with target hardware utilizations. It leverages on-device NPU (Neural Processing Unit) capabilities for efficient inference.
Github repository
The result demo application source code for the On-device YOLOv8 demo is prepared at the repository.
Implementation Guide
Prerequisites: Prepare YOLOv8 model and sample data
The YOLOv8 model
A sample input as a NumPy array
ZETIC.MLange module file
Android: zeticMLangeYoloV8.aar
iOS: zeticMLange.framework.zip for YOLOv8
Step 1: Generate ZETIC.MLange Model Key
Generate MLange Model Key with mlange_gen
Step 2: Implement ZETIC.MLangeModel
Android (Java):
For the project setup, please follow deploy to Android Studio page
iOS (Swift):
For the project setup, please follow deploy to XCode page
Step 3: YOLOv8 Image Feature Extractor
Android (Java)
iOS (Swift)
Step 4: Putting It All Together
Android (Java)
iOS (Swift)
Conclusion: YOLOv8 and On-Device AI - Innovation at the Edge and Limitless Potential
The combination of YOLOv8 models and on-device AI is revolutionizing computer vision technology not only on mobile devices but also on edge devices. This powerful synergy offers several key advantages:
Realization of Real-time Processing: The fast processing speed of YOLOv8 coupled with the immediate response capability of on-device AI enables real-time object detection and tracking.
Enhanced Privacy: As data never leaves the device, the security of sensitive information is significantly strengthened.
Offline Functionality: Advanced AI features can be used without an internet connection, ensuring reliable performance in any environment.
Energy Efficiency: On-device processing reduces overall energy consumption, extending battery life.
Edge Device Optimization: Lightweight versions of YOLOv8 operate efficiently on edge devices with limited computing power. This allows advanced AI functionalities to be implemented even on low-spec devices such as CCTV cameras or IoT sensors.
Utilization on Limited Chipsets: YOLOv8 maintains impressive accuracy and speed even on chipsets with limited performance. This enables its use in various fields such as smart home devices, wearables, and industrial sensors.
Development of Customized Solutions: Developers can easily create AI solutions tailored to various industries based on YOLOv8.
This combination of technologies opens up endless possibilities. For example:
Implementation of advanced multi-object tracking systems on low-spec CCTV
Image segmentation specialized for industrial IoT sensors
Real-time gesture recognition and emotion analysis on wearable devices
Providing innovative user experiences combining object recognition and augmented reality on smart home devices
The fusion of YOLOv8 and on-device AI goes beyond mere technological innovation; it's opening new possibilities across our daily lives and various industries. By enabling high-performance AI implementation even in resource-constrained environments, it is leading us into an era of AI-based solutions that are smarter, safer, and more efficient.
Do you have more questions? We welcome your thoughts and inquiries!
For More Information: If you need further details, please don't hesitate to reach out through ZETIC.ai's Contact Us.
Join Our Community: Want to share ideas with other developers? Join our Discord community and feel free to leave your comments!
Your participation can help shape the future of on-device AI. We look forward to meeting you in the exciting world of AI!